아두이노 보드에 이렇게 구성한다.
8번 포트를 아웃푹으로 지정
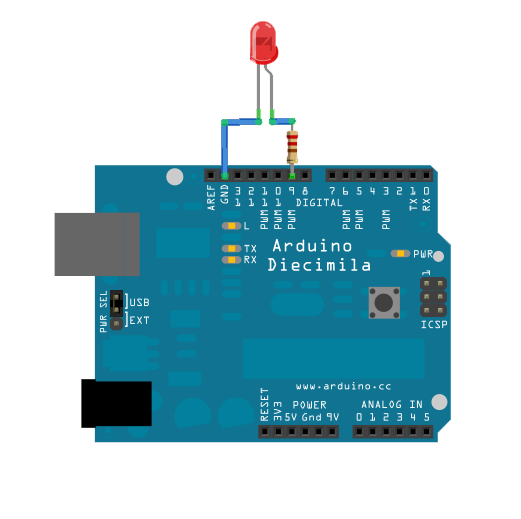
아두이노 소스
const int ledPin = 8; // the pin that the LED is attached to
void setup()
{
// initialize the serial communication:
Serial.begin(9600);
// initialize the ledPin as an output:
pinMode(ledPin, OUTPUT);
}
void loop() {
byte brightness;
// check if data has been sent from the computer:
if (Serial.available()) {
// read the most recent byte (which will be from 0 to 255):
brightness = Serial.read();
// set the brightness of the LED:
analogWrite(ledPin, brightness);
}
}
프로세싱 소스
(원 소스는 http://www.learningprocessing.com 에서 있으며, 그림 예제에서 일부를 수정해서 만들었음)
import processing.serial.*;
Serial port;
boolean button = false;
int x = 150;
int y = 150;
int w = 100;
int h = 75;
void setup() {
size(400,400);
// 디버그
println("Available serial ports:");
println(Serial.list());
// 내 pc 에는 Serial 내부 구조의 1번에 com3로 연결된 아두이노가 있다.
port = new Serial(this, Serial.list()[1], 9600);
}
void draw() {
if (button) {
background(255);
stroke(0);
} else {
background(0);
stroke(255);
}
fill(175);
rect(x,y,w,h);
}
void mousePressed() {
if (mouseX > x && mouseX < x+w && mouseY > y && mouseY < y+h) {
button = !button;
}
// 버튼의 값이 true이면 led을 끼고, false이면 led를 끈다.
if (button == true) {
// turn on led
port.write(255);
} else {
// turn off led
port.write(0);
}
}
여기서 약간 나는 머리를 썼다.
LED를 재미있게 만들었다. 원래 선물받은 제품인대, 꼬마 전구가 문제가 생겨서 못쓰고 있었다.
(사실 나는 "손에 잡히는 아두이노" 책에서 영감받아 나만의 재미있는 것을 만들려던 참이었다.)
꼬마 전구 대신 간단한 LED를 이용했다. 그리고 간단하게 테이프를 붙였다.
(. 원리는 무척 간단한데, 이런 제품을 구하기 가 어렵다는 게 있다. )
그리고 아두이노에 연결했다
처음에는 검은 바탕으로 있다. 꺼져있다.
가운데 버튼을 눌러주면 환하게 켜진다.
그렇게 복잡하지는 않지만, pc에서 아두이노를 다룬다는 것은 재미있다. 복잡한 프레임워크나 어려운 코드를 쓰지 않아도 내가 원하는 것을 할 수 있다니 매력이 있다.
흐흐.. 이제 점점 장난감으로 갈 것 같다.
'아두이노' 카테고리의 다른 글
프로세싱(processing) + 이클립스(연동) (1) | 2011.12.12 |
---|---|
프로세싱 언어가 자바 언어로 변환 (0) | 2011.12.09 |
[아두이노] 프로세싱(Processing) 과 아두이노(arduino)를 연동해보기 (3) | 2011.12.08 |
아두이노 Arduino 1.0 Release (0) | 2011.12.02 |
아두이노 (arduino) 에 리모콘 콘트롤러 (remote controller) 이용하여 적외선(ir) 테스트해보기 (0) | 2011.11.16 |